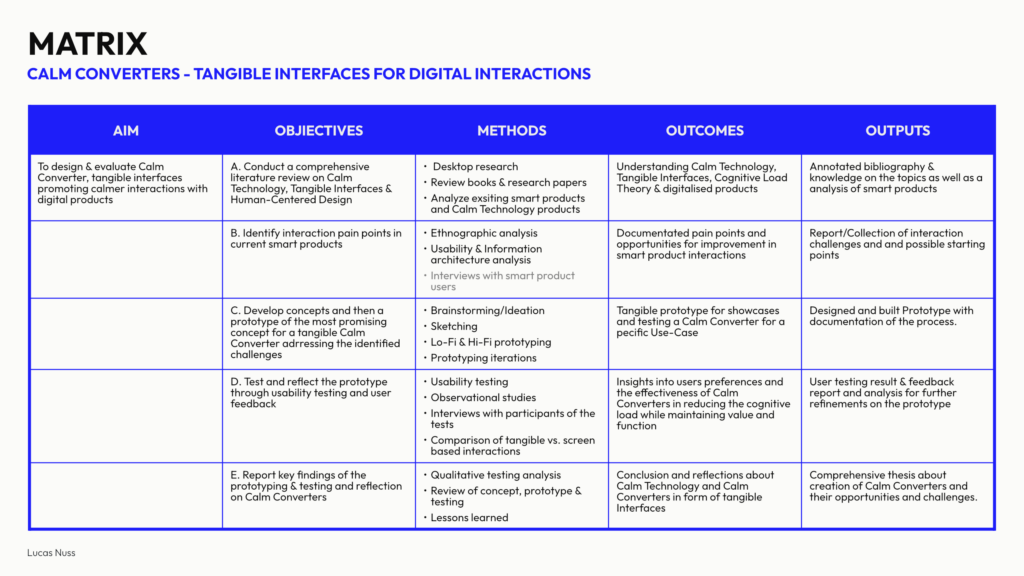
Master Matrix
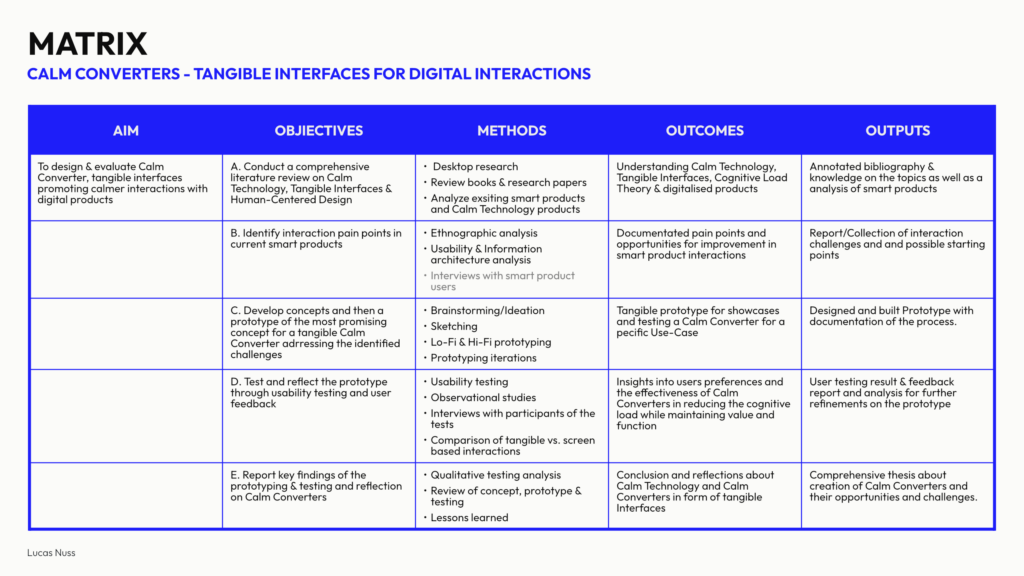
„Electric Dreams“ Kill All Others (Fernsehepisode 2018) – IMDb
For my final impulse blog post of the semester, I decided to re-watch one of my favourite episodes of the Philip K. Dick television series Electric Dreams, called Kill All Others, and analyse the role of technology and interfaces in this dystopia. The following is a short summary without spoiling too much, so you can still watch it yourself, which I highly recommend. The episode presents a future where mass media, consumerism and propaganda shape the perception of reality. It follows Philbert Noyce, a factory worker, in a world where a single political candidate dominates the media and spreads an ominous message: „Kill all the others“. While most citizens accept this statement without question, Philbert is disturbed. As he begins to notice disturbing details in his environment, such as the omnipresent holographic advertisements and growing social conformity, he realises that his scepticism makes him an outsider. His refusal to engage with voice-activated technology and his preference for tactile interactions highlight his resistance to blind conformity. But in a society where asking questions is dangerous, his refusal leads to his end. The episode explores themes of control through technology that are highly relevant to the study of Calm Technology and Tangible Interfaces. It presents an exaggerated version of our current digital landscape, offering valuable insights into the implications of technology in everyday interactions.
Consumerism
In Kill All Others, consumerism is driven by holographic advertisements that are directly linked to products, creating an illusion of authenticity. These holograms interact with consumers as if they have real emotions, making the experience feel personal and engaging. However, these interactions are entirely artificial and designed solely to manipulate and persuade. This highlights a major problem with modern technology: the replacement of real human connections with simulated digital experiences.
Calm Technology aims to reduce this overwhelming presence of digital distractions by integrating technology more seamlessly into everyday life. Instead of bombarding users with aggressive notifications, advertising and emotional manipulation, Calm Technology prioritises subtle and meaningful interactions. Tangible interfaces could play a role in countering digital consumerism by encouraging real, physical engagement with products and services rather than relying on artificial digital interactions. For example, instead of a holographic assistant selling a product, a Tangible Interface could allow users to explore the features of an item through touch, movement or changes in texture, encouraging a more mindful and intentional relationship with technology.
Tactility
In the episode, most of the world operates via voice-controlled routers, making interactions seamless but also impersonal. Philbert, however, continues to use tangible buttons, setting him apart from the majority. This reflects a critical discussion in interaction design: while voice control and other intangible interfaces offer convenience, they often remove the physical engagement that strengthens a user’s connection with technology. Tangible interfaces offer an alternative by maintaining the physicality of interaction. They provide feedback that is not only visual or auditory, but also tactile, giving users a greater sense of control. In the case of Kill All Others, Philbert’s reliance on tangible buttons can be seen as an act of defiance. He values the certainty that comes with physical interaction and resists the abstract nature of voice commands, which can be easily manipulated or misunderstood. This is closely related to Calm Technology, as tangible interactions allow users to remain in control without being overwhelmed by digital noise. A future designed with Calm Technology and Tangible Interfaces in mind could balance the convenience of voice control with the reliability of touch-based interactions. For example, rather than relying solely on voice assistants, smart home devices could incorporate physical interfaces that provide intuitive and non-intrusive ways to interact with technology.
Reflection
One of the most striking ideas in Kill All Others is how Philbert is labelled „too tactile“, as if his preference for physical interaction is a flaw. His scepticism and reliance on touch makes him resistant to the influence of the media, as tactile perception is harder to fool. This reflects an important advantage of tangible interaction: it provides a direct and reliable form of engagement with technology.
In a world where digital information can be manipulated, a focus on tactile interaction could act as a safeguard against misinformation. For example, in Internet of Things (IoT) design, physical objects that translate digital messages into tangible experiences could help users process information in a more grounded and thoughtful way. Rather than overwhelming users with constant digital alerts, these interfaces could use subtle physical changes, such as changes in temperature or texture, to convey information. This would allow for a calmer, more meaningful interaction with technology, reducing the risk of mindless engagement and manipulation.
As is often the case, fiction can be a great source of inspiration and warning for many things in our time. So if you haven’t seen Philip K. Dick’s Electric Dreams series, I would strongly recommend that you do, because it visualises important issues and makes you think about what our future should look like.
Project Zanzibar: A Portable and Flexible Tangible Interaction Platform
After refreshing my research on Calm Technology, I wanted to look at Tangible Interfaces for my next blog post, as it is the second component of my soon to be started Master’s thesis. While researching for an impulse like an event or presentation, I found one of the most exciting tangible interface developments I have seen so far. Microsoft’s Project Zanzibar. So I decided to explore and get to know the project instead. This innovative research project presents a flexible, rollable mat that enables seamless interaction between the physical and digital worlds using touch, gestures and tagged objects. It creates what I believe to be a calm, tangible interface without losing the digital benefits of smart products and without being intrusive or demanding.
The Project
Project Zanzibar is a tangible interaction platform developed by Microsoft Research. At its core is a flexible mat embedded with a Near Field Communication (NFC) system and capacitive sensing technology. This enables the mat to track touch, recognise gestures and interact with physical objects placed on it. The mat is designed to be portable, with a rollable form factor that makes it easy to transport and use in different contexts. Unlike traditional tabletop interfaces that rely on optical tracking and projection, Zanzibar integrates NFC and capacitive sensing to enable real-time object recognition and manipulation without the constraints of fixed installations. This makes it a promising tool for game design, education and creative storytelling, offering new possibilities for tangible interaction.
The Zanzibar mat identifies and tracks objects using NFC tags, which are simple, low-cost stickers attached to physical objects. These tags store unique identifiers that allow the mat to recognise specific objects and their movements. In addition, the capacitive sensing technology enables multi-touch interactions and hover gestures, enhancing the way users can interact with digital content through physical manipulation. One of the unique features of Zanzibar is its ability to sense object orientation and stacking. By using special NFC tag designs with metallic properties, the mat can detect the rotation of an object. Stacking tags allow Zanzibar to determine the order of stacked objects, making it ideal for applications such as board games or layered data visualisation.
One of the biggest challenges in interaction design is finding ways to physical and digital experiences seamlessly. Zanzibar demonstrates a compelling approach by enabling natural interactions with everyday objects, making digital experiences more intuitive and engaging. By supporting energy harvesting NFC tags, the platform enables more advanced interactions. For example, interactive tags can be used to light up LEDs or trigger small actuators, creating dynamic and responsive tangible objects. These features could be particularly useful in education, where physical engagement improves learning outcomes, or in IoT applications, where physical controls could interact with intelligent environments.
Applications
Project Zanzibar has been explored in a number of interactive applications, particularly in the gaming and education sectors. For example, Pirate Toybox is a game where physical toy figures placed on the mat trigger corresponding digital dialogues and actions. The system uses audio feedback to create immersive, screen-free gaming experiences. Another exciting use case is Movie Maker, an application aimed at children aged 5-12. This tool allows children to tell stories by manipulating physical toys and props on the mat, which then control digital avatars on a connected screen. This provides an intuitive way for children to explore storytelling and animation through tangible interactions. Zanzibar has also been tested in Augmented Reality (AR) applications. In AR Tower Defence, the mat is used in combination with a Microsoft HoloLens device to create a hybrid AR gaming experience. Players use tangible objects to control characters and place defences, while viewing digital overlays through the AR headset.
Reflection
As I will be focusing on tangible interfaces for notification interactions and smart products in my research and master’s thesis on Calm Technology, Project Zanzibar serves as an inspiration for designing more seamless and ambient interactions. The mat’s ability to integrate digital notifications through tangible objects provides a unique opportunity to explore alternative ways of interacting with digital information beyond screens and traditional input methods. This is exactly what I am trying to achieve with the practical part of my master’s thesis. Imagine, for example, a home automation system that uses tangible objects on a Zanzibar-like surface to control lighting, temperature or music in an intuitive, non-intrusive way. It shows that with the technology we already have, we are no longer tied to screen-based and disruptive products, and that is what we should be aiming for to create a future where digitality and reality work in balance, respecting human needs and boundaries once again.
Keynote: Calm Technology: Design for the Next 50 Billion Things – Amber Case
For my next blog post, I decided to dive back into the topic of Calm Technology. To do this I watched Amber Case’s keynote at the Embedded Linux Conference: “ Calm Technology: Design for the Next 50 Billion Things“. Amber Case herself is one of the leading researchers in the field of Calm Technology and has not only written a book on the subject but also recently founded the Calm Tech Institute. Her talk refreshed much of what I have been researching over the last two semesters about calm technology, but also gave me a new perspective or two and challenged me to keep exploring this, in my opinion, most important and fascinating design philosophy.
The Talk
Case began by introducing the concept of modern humans as ‚cyborgs‘ – not in the science fiction sense, but as beings who use external tools to adapt to new environments. This concept immediately resonated with my interaction design studies, as it highlights how deeply technology has become integrated into our daily lives. However, as Case pointed out, the current state of this integration is far from ideal.
The core problem she identified is what she calls ‚interruptive technology‘ – devices and systems that constantly demand our attention, often unnecessarily. From duplicated smartwatch notifications to ’smart‘ fridges that create more problems than they solve, our current technological landscape seems designed to interrupt rather than support. As someone with an interest in calm technology, this observation hit close to home, as I’ve often wondered about the real value these ’smart‘ additions bring to users‘ lives, and whether they need to be as dictating as they are, or if there are other ways. The solution, according to Case, lies in “ Calm Technology“ – a concept developed at Xerox PARC by Mark Weiser and John Seely Brown. The principle is simple: technology should require the minimum amount of attention and remain in the background until needed. This approach feels quite logical and makes perfect sense from a human-centred design perspective, but in our current context of attention-hungry devices it is revolutionary.
One of the most valuable insights for my future work was Case’s emphasis on peripheral attention and ambient awareness. She explained how technology should be designed to operate in our peripheral awareness, similar to how we notice changes in our environment without actively focusing on them. The example of a tea kettle illustrates this perfectly – it doesn’t require constant attention, but communicates effectively when needed.
The presentation also highlighted the importance of using the minimum amount of technology necessary to solve a problem. This principle particularly challenges current trends in interaction design, where we often add features because we can, not because we should. Case’s example of over-complicated smart home systems versus simple, reliable mechanical solutions made me even more certain that the topic I had chosen to research was the right way to go. Perhaps most importantly, Case emphasised the need to consider social norms and the ‚metabolism rate‘ at which people can adapt to new technologies. This insight is crucial to my Masters, as it suggests that successful interaction design isn’t just about creating innovative solutions, but also understanding how those solutions fit into existing social contexts and behaviours. The concept of ‚kairos time‘ versus ‚chronos time‘ provided a powerful framework for thinking about the impact of technology on our experience of time. As interaction designers, we should strive to create solutions that respect and enable moments of flow and meaningful engagement, rather than constantly fragmenting users‘ attention.
Reflection
This keynote reminded me of how important Calm Technology can be and is for interaction design. It has shown me that perhaps the most sophisticated design solutions are those that know when to step back, remain invisible, and only emerge when truly needed. This is in line with one of my beliefs that products should not scream for attention, but rather receive it because it is useful, practical or wanted. As I continue my studies and begin my Master’s thesis, I will take these principles of Calm Technology with me and explore them to create designs that enhance human capabilities without demanding unnecessary attention.
The challenge for me as an interaction designer is clear: how do we create technology that supports without overwhelming, assists without interrupting, and enhances without dominating? Case’s presentation provided valuable guidance on how to address these questions in my future work and master’s thesis.
As an industrial designer now studying interaction design for my next impulse, I finally decided to watch the film Rams (2018), a documentary directed by Gary Hustwit about Dieter Rams, one of the most influential industrial designers of the 20th century. It was an enlightening experience, and it made me think deeply about the philosophy behind good design and how it applies to the digital world I work in today. Dieter Rams is best known for his work at Braun and Vitsœ, where he designed some of the most iconic products of the 20th century, including radios, coffee machines and shelving systems. His creations were not only visually attractive, but also highly functional. What makes the documentary special is its focus on Ram’s design philosophy, rather than just his products. It explores his principles of ‚less but better‘ and how his work relates to wider ideas of sustainability and responsibility in design.
The Movie
One of the central themes of the documentary is Rams‘ famous „Ten Principles of Good Design“. These principles define what Rams believes makes a design truly great. They emphasise qualities such as innovation, usability, honesty and environmental friendliness. While all ten principles are equally important, a few stood out to me in relation to interaction design and the digital world:
Although Rams worked primarily in industrial design, his principles are incredibly relevant to the field of interaction design. For example, his focus on usability and clarity is very much in line with the concept of user-centred design. Watching the documentary made me think about how I could apply his principles to my own projects, especially as someone who works at the intersection of digital products and physical interfaces.
In the documentary, Rams talks about designing for people rather than trends. This approach seems particularly important in today’s world, where design often prioritises novelty over usability. For example, many apps and devices are packed with unnecessary features that make them harder to use rather than easier. Ram’s philosophy reminds us to put the user first and focus on creating designs that truly improve people’s lives.
Another key takeaway for me was Rams‘ commitment to designing with honesty. He believed that a product should not pretend to be something it is not. In interaction design, this could mean avoiding deceptive patterns like fake loading screens or manipulative notifications. Instead, we should aim to build trust with users by being transparent and respectful.
Conclusion
As someone who researched Calm Technologies for my Master’s thesis, I was particularly inspired by the idea of unobtrusiveness in Rams‘ work. Calm Technology is about creating systems and interfaces that exist in the background and only demand attention when needed. This concept fits perfectly with Rams‘ belief that good design is quiet and unobtrusive.
For example, Rams‘ designs often had a timeless quality. They didn’t rely on trends or gimmicks to stay relevant. In the same way, Calm Technology encourages us to move away from designs that constantly compete for attention. Instead, it asks us to create products that fit seamlessly into users‘ lives, enhancing their experience without overwhelming them.
Reflection
One of the most striking parts of the documentary was when Rams talked about his regrets about contributing to a world of over-consumption. He expressed concern that his designs, while successful, may have encouraged people to buy more than they needed. This reflection is a powerful reminder of the responsibility we have as designers. Whether we create physical products or digital interfaces, our work shapes how people interact with the world.
In my mind, Rams (2018) was not just a documentary about a designer, it was a call to action. It challenged me to think more critically about the impact of my designs and to aim for simplicity, honesty and sustainability in everything I create. As I continue my studies and work on my thesis, I hope to carry Rams‘ principles even more with me then before and use them as a guide to create meaningful and responsible designs.
I highly recommend watching Rams if you are a designer – or simply someone interested in the role design plays in our lives.
ProtoPie 101 Crash Course | ProtoPie School
After completing the first half of the ProtoPie Crash Course, I was motivated to dive into the second half because of the quick learning and skills I had already gained in the first part of the course. With three more lessons this time about advanced techniques, I gained a deeper understanding of ProtoPie’s capabilities. The content this time was Conditional Logic and Triggers, Variables and Functions & a Wrap Up to summarise and review all the learning from the whole course.
Lesson 4: Conditional Logic and Triggers
The fourth lesson introduced me to conditional logic and advanced triggers. These features allowed me to create interactions that responded intelligently to user inputs. This was a significant step up from the basic interactions we learned earlier.
Conditional Logic
We started by creating a password validation interaction using conditionals. This exercise showed me how to add logic to prototypes without needing to write a single line of code. By setting conditions, I was able to create a prototype that checked whether a password met specific criteria and provided real-time feedback to the user.
Chain and Range Triggers
Next, we explored the Chain Trigger, which is used for creating navigation aids. I designed an interaction where tapping on different sections of a menu smoothly scrolled to the corresponding part of the page. The Range Trigger was another great too which I used to create an auto-play video carousel that responded dynamically as the user scrolled. Both triggers added a new layer of sophistication to my prototypes.
Lesson 5: Variables, Functions, and Components
The fifth lesson was all about harnessing the full power of ProtoPie by using variables, functions, and components. These features gave me access to the possibilities of creating complex, yet manageable, prototypes.
Variables and Functions
I started by learning how to use variables and formulas to store and manipulate data within a prototype. This was a game-changer for me, as it allowed for dynamic interactions. For example, I created a camera focus point interaction where users could tap anywhere on the screen to adjust the focus dynamically. Using variables made the interaction feel incredibly realistic.
Custom Greetings and Smart Light Control
Next, I built a customized greeting interaction that displayed the user’s name based on their input. This feature demonstrated how ProtoPie could personalize experiences. We also designed a smart light control prototype where users could adjust the brightness and color of a light bulb. This exercise showcased how ProtoPie could simulate IoT interactions effectively.
Multi-Screen Smart Home Control
The highlight of this lesson was creating a multi-screen smart home control interface. By using components and the Send & Receive feature, we linked multiple screens together seamlessly. This exercise emphasized the importance of reusability and organization in prototyping complex systems.
Lesson 6: Wrapping Up and Moving Forward
The final lesson was a wrap-up session that consolidated everything we had learned throughout the course. It included a knowledge exam, which tested our understanding of ProtoPie’s features. I was happy to pass the exam and receive my certificate of the crash course in Protopie.
Helpful Resources
Before the course ended, we were provided with a lot of resources to continue our ProtoPie journey. These included detailed documentation, community forums, and example projects. Knowing that I have these resources to look up gives me confidence to tackle even the most challenging prototyping tasks in the future.
Reflections
The second half of the ProtoPie crash course, like the first, was interesting and full of useful skills and possibilities for future prototypes. It opened my mind to not only think about how to design interactions that are both functional and intuitive, but also that I am now able to test and prototype them myself. The hands-on exercises, as in the first part, allowed me to experiment with the more advanced features and gain practical experience, which, as I said before, is the only way I really learn, by trying things and doing them. By the end of the course, I felt equipped to create prototypes that go beyond static designs and truly mimic real-world interactions. Because ProtoPie’s is so easy to use, I think it will be my go-to tool for prototyping. It is also a good element for my Master’s thesis, in which I plan to connect the analogue and digital worlds in a calmer way by creating new ways of interacting between them. As I plan to build and test a physical prototype in the thesis, I will most likely need some sort of digital layer, which I now feel able to realise, or at least build a mock-up of.
ProtoPie 101 Crash Course | ProtoPie School
For this semester and next, we have been given the opportunity to use Protopie with a full licence as part of our studies. Because this was introduced in a subject where we could choose the topic, we wanted to work on ourselves, and the topic I chose was a group project to further develop a game we made in the first semester of the Masters. I decided to use two of my impulse blog posts to learn how to use and prototype with Protopie. Fortunately, Protopie offers a comprehensive crash course, divided into six lessons, to learn and master many of the possibilities it offers. As the course is quite extensive, I have split it into two parts, each covering three lessons of the course. So here is the first half of the course on the basics, interactive transitions & sensor-based interactions.
Lesson 1: An Introduction to ProtoPie’s Core Features
The course started off with a comprehensive introduction to ProtoPie. The first lesson covered the tool’s three main purposes: to create, test, and share prototypes. This was perfect for me, as I’d only ever worked with Figmas prototyping tools before. ProtoPie promised to enable more dynamic and realistic interactions.
Creating Prototypes
It started with learning how to set up our projects. The process was straightforward. Once the project was ready, we explored how to seamlessly import designs from tools such as Figma, Adobe XD or Sketch. Next, we were introduced to the basic features of ProtoPie. I learned how to create interactions by simply dragging and dropping elements. The interface was intuitive, even for someone with limited experience of advanced prototyping. Creating interactions felt like building with digital Lego – any action or trigger could be linked to create a seamless process.
Testing and Sharing
Once my prototype was ready, the next step was to test and share it. ProtoPie allows us to view our prototypes directly on devices such as smartphones and tablets, which made them tangible. I could see how the designs would work in real-life scenarios. Sharing was just as easy. I uploaded my project to the ProtoPie Cloud, which made it easy to collaborate with others. Another good feature is the interaction recordings. These allow you to document specific interactions. ProtoPie also has the functionality of Interaction Libraries, which allows teams to standardise design components. This can certainly save a lot of time on larger projects.
Lesson 2: Exploring Interactive Transitions
In the second lesson, it was time for hands-on with creating various types of interactions.
Screen Transitions
It started by teaching how to prototype automatic, semi-automatic, and fully custom screen transitions. I particularly enjoyed working on custom transitions because they allowed me to design interactions tailored to specific design case.
Scrolling and Paging
Next, the course dived into scrolling and paging interactions. I had always struggled to make scrolling interactions look good or useful in Figma. In ProtoPie the results were realistic, exactly like the scrolling you’d expect in a native app.
Sliding Menus
The last part of this lesson was designing sliding menus. We explored three different ways to create them, ranging from simple swiping gestures to more complex interactions that combined multiple triggers.
Lesson 3: Sensor-Aided Interactions
The third lesson took ProtoPie’s capabilities to the next level by introducing sensor-aided interactions. This feature truly sets ProtoPie apart from other prototyping tools because it enables designers to use device sensors without needing any coding knowledge.
Using Device Sensors
The workshop started with an introduction to using a phone’s camera in prototypes. I created interactions where the camera’s feed became part of the design. This was particularly useful for scenarios like augmented reality apps or interactive tutorials.
Input Fields and Native Keyboards
Next, the course explored prototyping with input fields and native keyboards. This feature was a pleasant surprise, as it allowed me to create realistic forms and search bars that behaved just like the ones in real apps. I can already see how this could improve user testing sessions, as participants would interact with the prototypes in a natural way.
Voice Interactions
The final part of this lesson focused on voice interactions. ProtoPie made it easy to incorporate voice commands and responses into prototypes. This feature opened endless possibilities for designing interfaces for voice-activated devices or accessibility features. I was amazed at how simple it was to implement this functionality.
Reflections
The first three lessons of the ProtoPie crash course already showed a lot of possibilities in prototyping. Each lesson built on the previous one, gradually introducing more complex features. I appreciated the hands-on approach, as it allowed me to apply what I learned immediately, which is the best way for me to learn and retain things.
A(R)dventure – CoSA | CoSA – Center of Science Activities
For my second impusle blog post I decided to visit an exhibition at the CoSa Museum in Graz together with my fellow student Vinzenz. The A(R)dventure 3: Habitat Red 6. This installation combines augmented reality through Microsoft’s HoloLens with physical interactions, creating an experience that was not only fun & engaging, but also made me think about climate change and the future possibilities of AR technology in the field of interaction design.
The Visit
When I arrived, I was greeted by an enthusiastic member of the Project, who explained the concept thoroughly and helped me get started using the HoloLens. The adventure began as I followed H.I.G.G.S., a digital drone guide, through a fascinating time vortex that took me digitally through the lenses and physically by foot to a habitat on another planet in need of maintenance and care.
The first task was to manage the atmosphere control system. What made this interaction particularly interesting was the combination of physical valve controls with digital displays. As I turned the real knobs, I could see the atmospheric parameters changing on virtual graphs floating in front of my eyes. This immediate feedback loop between physical action and digital response created a natural and intuitive experience.
The next task took me to the power management system, where I found myself adjusting solar panels using a physical turning wheel. Through the HoloLens, I could see a window showing the actual panels moving outside the habitat, demonstrating how my actions directly affected the station’s power supply. This mix of tangible control and virtual feedback made adjusting something as small as a solar panel very important and interesting, and made me realise that solar power is very location dependent.
One of the less interactive tasks was plant pollination. Starting in a physical test chamber, I learned about the process on a small scale through an animation, before seeing it applied to a larger virtual plantation visible through digital windows. This move from small scale learning to larger scale implementation helped by first understanding the concept and then seeing it applied and its effects on the displayed plantation biosphere.
After pollination, the water filtration system needed some attention. Using a physical joystick, I operated a virtual crane to service the water purification system. Replacing an old filter with a new one. The amount of work required to have clean water was highlighted by this task, showing the importance of clean water, something we often take for granted on Earth.
Perhaps the most tactile experience was selecting materials for heat shield repairs. I was able to physically touch and examine different materials while receiving digital information about their properties and suitability for the repair job. This combination of sensory feedback and augmented information created a powerful learning experience about materials science.
The final task involved fine-tuning the habitat’s environmental controls using spring-loaded dials. As I adjusted humidity and other parameters, the digital graphs responded in real time and, importantly, these changes, like all other changes, persisted in the virtual environment, creating an immersive feeling that demonstrated the lasting impact of my actions.
Conclusion
The exhibition successfully communicated its underlying message about the Earth’s ecosystem without hitting you on the head, which is a difficult line to walk. By asking visitors to maintain artificial living conditions, it effectively illustrated the complexity of our planet’s natural systems, which we often take for granted. Each challenge in maintaining the habitat served as a reminder of the Earth’s perfectly balanced ecosystem. All in all, I found it a great experience and would advise anyone to try something similar if they have the opportunity.
Reflection
What struck me most about this experience was how it challenged my previous assumptions about calm technology. As someone researching this area for my Master’s thesis, I had initially leaned towards purely analogue solutions and viewed AR and VR as potentially intrusive technologies. However, Habitat Red 6 demonstrated that a thoughtful combination of physical and digital interactions can create experiences that are both engaging and intuitive. The digital layer added depth and dynamics to the physical interactions, while the tangible elements grounded the experience in reality. This hybrid approach retained the benefits of physical feedback, while using digital technology to provide additional context and visualisation that would not be possible with purely analogue interfaces.
This experience significantly influenced my perspective on interaction design. Instead of seeing analogue and digital as opposing approaches, I now see the potential in combining tangible objects with digital layers of information to create interfaces that offer the best of both worlds. This insight will definitely influence the direction of my Master’s research, opening up new possibilities for designing more intuitive and meaningful interactions.
Over the past two semesters of researching Calm Technology, I have begun to focus more and more on Human-Computer Interaction (HCI) and how to align it with the principles of Calm Technology to combat the negative effects of the digitalisation of our environment. My goal is to create or change the converter of digital interactions to make them materialise the digital in a more natural way. In my opinion, this can only be done by changing the way, mode and nature of the interactions we currently have with the digital world. So I will try to get impulses in different human-computer interactions and how a calmer materialisation of the digital can be achieved. My first impulse for this is the Klanglicht festival in Graz, as it also partly explores ways of communicating complex information, feelings or stories with the reduced methods of only using sound and light. This happened from two perspectives, one as a visitor and one as a participant in the installation Spektrum of the FH Joanneum.
Klanglicht
From the perspective of a visitor to Klanglicht, it was interesting to see in how many different ways different artists were able to communicate or convey sometimes quite complex stories, emotions or meanings without using traditional storytelling media such as screen or spoken or written text. Only by using sound, light and in some cases movement were they able to convey all this in a clear way. This was achieved by abstracting all the elements of communication between the installation and the visitor and placing them in the right context. Calm Technology aims to do just that, moving away from attention-grabbing push notifications and messages to more natural and contextual interactions that are less overwhelming for the user. This can be achieved by using movement or lights in the periphery of the vision, or by using sounds that convey secondary information in context. This was demonstrated in a more artistic but nonetheless true way by the projects exhibited at Klanglicht. One installation called ENDERS / surface by Boris Acket was particularly inspiring on this theme. The way in which he achieved an almost natural and very calming environment using only fairly technical elements such as motors and stage lighting, and using these elements to create an interplay of shadow and light with subtle movement, was an impressive example of how technology can be used to create something calm and detached from its digital parts. The unpredictable but harmonious movement of the fabric mirrors natural phenomena such as the ripple of water or the wind through grass, creating a sense of calm and connection with the environment. The mirroring of familiar, natural patterns can be a key factor in making human-computer interactions more ambient and enjoyable. By orienting these interactions around the rhythms and behaviours of our natural world, rather than the rigid structures of digital systems, we can create ambient interaction that feel more intuitive and calming. By using our learned understanding of natural behaviours instead of us having to learn new and unnatural patterns and behaviours.
Langnicht
As participants the impulse of Klanglicht was more practical than as visitors. Together with all four majors of our study programme (Communication, Media, Sound & Interaction) we had the opportunity to realise our own sound & light installation under the theme Spektrum in the Antoniuskirche in Graz. The first challenge was to set up the installation inside the church, where we were not allowed to change anything and everything had to be self-supporting as the building is a listed building. But after a few rounds of trial and error and many hours of work, we managed to create a rather impressive and complex installation. As shown in the video below. This first challenge taught me a lot about how to structure projects, how to prioritise tasks and that the more work you do in the background, the smoother and better the experience at the front. The second challenge was to create a three-minute sound and light show to be played on the installation, now in smaller interdisciplinary groups of 5-6 students. Our chosen story for this show was the specter of nature and technology, how they contradict each other, but can also find common ground in a utopian solar punk vision. To convey this message we had only the medium of spatial sound and the construction of LED pixel rails with individual LED dots rather than an LED screen. The learning experience here was how to abstract a message so that it would only work if you heard sounds and saw light dots glowing in an abstract pattern, without losing any of its meaning. In the end, as you can see in the teaser below, we achieved this in some way. This showed me how difficult or much work it is to reduce interaction elements without losing information or meaning in the process. It ended up being a very relevant exercise for me. Because in order to make interactions more ambient, they should follow this abstraction theme.
Author: Qian Ye
Title: Natural Interface in Mixed-Reality
Publiaction Year: 2020
Institution: Kunstuniversität Linz
Study Programme: Interface Cultures
Source: NATURAL INTERFACE IN MIXED-REALITY (The University of Art and Design Linz Phaidra – o:2046)
This thesis was chosen for my analysis because the field of Tangible and Natural Interfaces has a lot of overlap with the topic of Calm Technology by making human-computer interaction (HCI) smoother and less digital. What is ultimately one of the key points why I am interested in Calm Technology is to change technology to interact with us on our terms, rather than us having to adapt to technology.
Level of Design
The overall aesthetics of the thesis is clear, clean and well structured. It is easy to read and follow the line of thought. The content focuses on mixed reality interfaces that integrate natural elements for human-computer interaction (HCI). It explores Tangible User Interfaces (TUIs) and includes extensive theoretical research combined with artistic practice, showing a deep understanding of interface development.
Degree of Innovation
The thesis presents innovative ideas for merging physical and virtual worlds through mixed reality. In particular, the author proposes new ways of creating „natural“ interfaces, especially by using elements of nature. The inclusion of projects such as „TRIALITY“ and „Water Instrument“ adds a hands-on, experimental dimension, showing the practical side of the theoretical part.
Independence
The author demonstrates independence in research and design execution. The projects and experimental frameworks presented, such as the ‚PAPERPAL‘ educational interface for children, show a high level of self-directed exploration, especially in the development of unique interfaces.
Outline And Structure
The thesis is clearly structured. It begins with an introduction, followed by theoretical discussions, practical applications and concludes with future perspectives. The reader is directed through theory, artistic practice and contextual discussions in a coherent structure.
Degree of Communication
The thesis is effectively communicated, with well-written ideas and clear transitions between chapters. The author has a clear grasp of technical language and can express complex concepts concisely, making it easy to read and understand without oversimplification.
Scope of the Work
The scope is comprehensive, covering both theoretical and practical aspects of Mixed Reality, TUIs and educational applications. It addresses broader themes in interactive art while exploring specific approaches for children and natural elements, demonstrating a balanced approach between depth and width. All in all, a topic and work that meets the requirements of an academic Master’s thesis.
Orthography and Accuracy
The thesis appears to have been carefully proofread, maintaining high standards of spelling and accuracy throughout. The language is professional, with no noticeable errors, suggesting careful editing and attention to linguistic precision.
Literature
The literature review is thorough, with references to foundational HCI and interface design theories and pioneers such as Donald Norman and Mark Weiser. With a total of 92 different references, ranging from current to older but not obsolete resources, the author establishes a solid theoretical framework that supports the foundation of the thesis.
*All texts have been paraphrased using DeepLWrite.